Last.fm API
I recently recovered my Last.fm account, which was one of the top music services before Spotify or streaming in general. It says my account has been around since 2010, thus the cringy username xthekingisdeadx
. My profile picture and username still stay the same.
I want to retrieve my Last.fm data, particularly from my top artists and albums I have listened to for the past week or month. So I could share it somewhere.
I am going to write my script using Go. I started this project in Python but encountered a couple of errors and I am just not in the mood to debug or solve them. Weekend projects should be fun, no?
GET data
package main
import "fmt"
import "net/http"
func main() {
response, error := http.Get("https://ws.audioscrobbler.com/2.0/?method=user.gettopalbums&user=xthekingisdeadx&api_key=[api-key-here]&format=json&period=7day")
if error != nil {
}
defer response.Body.Close()
body, error := ioutil.ReadAll(response.Body)
fmt.Println(string(body))
}
So far so good, script compiled. Now I can retrieve the data, but no parsing yet. Time to Google, “golang parse JSON”.
JSON parsing
package main
import "fmt"
import "net/http"
import "io/ioutil"
import "encoding/json"
func main() {
response, error := http.Get("https://ws.audioscrobbler.com/2.0/?method=user.gettopalbums&user=xthekingisdeadx&api_key=[api-key-here]&format=json&period=7day")
if error != nil {
}
defer response.Body.Close()
body, error := ioutil.ReadAll(response.Body)
// fmt.Println(string(body))
var jsonResponse Response
json.Unmarshal(body, &jsonResponse)
var albums = jsonResponse.TopAlbums.Album
for _, album := range albums {
fmt.Println(album.Artist.Name, ":", album.PlayCount)
}
}
type Response struct {
TopAlbums TopAlbums `json:"topalbums"`
}
type TopAlbums struct {
Album []Album `json:album`
}
type Album struct {
Artist Artist `json:"artist"`
Image []Image `json:"image"`
PlayCount string
}
type Artist struct {
ArtistUrl string `json:"url"`
Name string
}
type Image struct {
Size string `json:"size"`
Text string `json:"#text"`
}
GUI Toolkit
Since I only have very minimal experience with the Go language, I am not sure what my next steps are. Either, I will go with the GUI toolkit route or image manipulation.
Switching to SwiftUI
After researching Go UI frameworks, I eventually decided to use what I am currently familiar and, that is SwiftUI. Another reason is the sad state of native UI frameworks using the Go language because it seems they aren’t many options available. I don’t want to use cross-platforms or UI frameworks using web technologies for this is just a simple project.
One hour later
Using LazyHGrid
, this is what it looks like now.
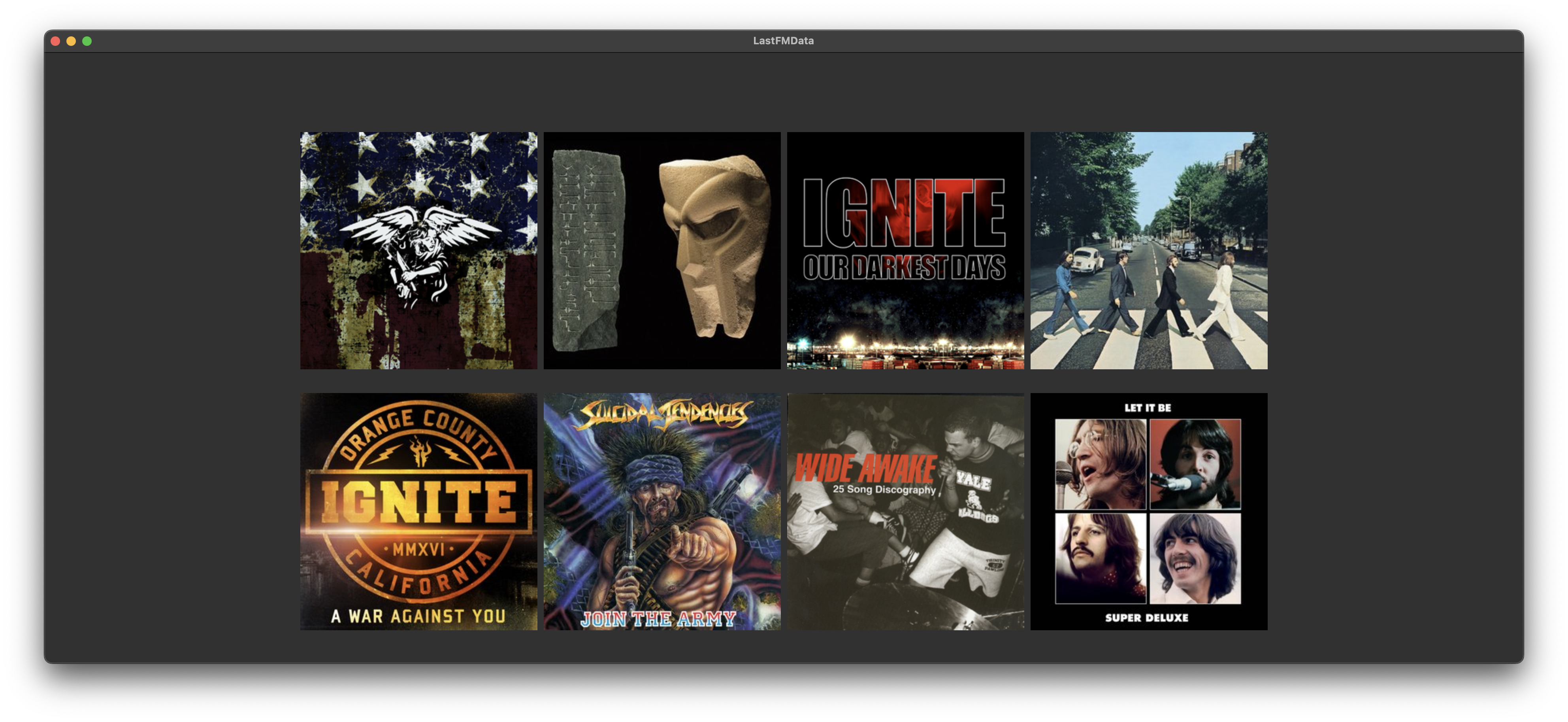
Next
My next step would be to be able to export this whole View
into an image.
Source Code
Code can be found here: https://github.com/lawgimenez/lastfmdata